Input/output and file formats¶
Load data into python¶
Import nibabel as nib (into a jupyter notebook)
Import NiftiMasker and MultiNiftiMasker of nilearn: from nilearn.input_data import NiftiMasker , MultiNiftiMasker
Load your mask of your region-of-interest as well as your epi-data into the jupyter notebook in order to get the voxels extracted of your region-of-interest for each of your epi-images.
def load_epi_data(sub, ses, task):
# Load MRI file
epi_in = os.path.join(data_dir, sub, ses, 'func', "%s_%s_task-%s_space-MNI152NLin2009cAsym_desc-preproc_bold.nii.gz" % (sub, ses, task))
epi_data = nib.load(epi_in)
print("Loading data from %s" % (epi_in))
return epi_data
def load_mniroi_mask(ROI_name):
mnimaskdir = os.path.join(mnirois_dir)
# load the mask
maskfile = os.path.join(mnimaskdir, "%s.nii" % (ROI_name))
mask = nib.load(maskfile)
print("Loaded %s mask" % (ROI_name))
return mask
If you want to open the actual image then add get_data() or get_fdata(), e.g.:
mask.get_data()
To then extract the voxels of your mask from your loaded epi-data:
nifti_masker = NiftiMasker(mask_img=mask)
maskedData = nifti_masker.fit_transform(epi_data)
If you want to high pass filter and clean data from confounds (e.g. motion, white matter, csf, etc.). The confounds_file is the file with the confounds you want to use that you can select from the many confounds that fmriprep outputs.
nifti_masker = NiftiMasker(mask_img=mask, high_pass=1/128, t_r=1.5)
maskedData = nifti_masker.fit_transform(epi_data, confounds=confound_file)
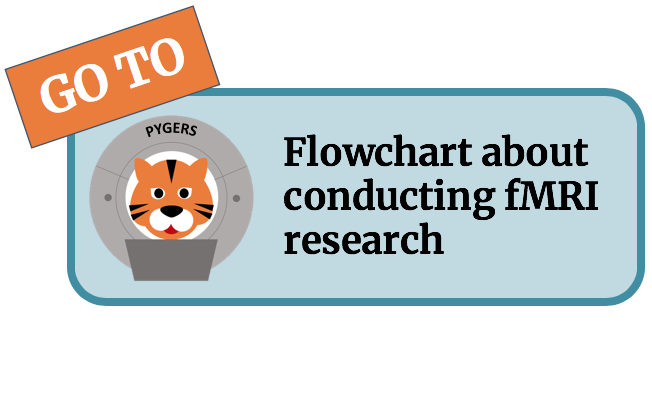